In a given grid, each cell can have one of three values:
- the value
0
representing an empty cell; - the value
1
representing a fresh orange; - the value
2
representing a rotten orange.
Every minute, any fresh orange that is adjacent (4-directionally) to a rotten orange becomes rotten.
Return the minimum number of minutes that must elapse until no cell has a fresh orange. If this is impossible, return -1
instead.
Example 1:
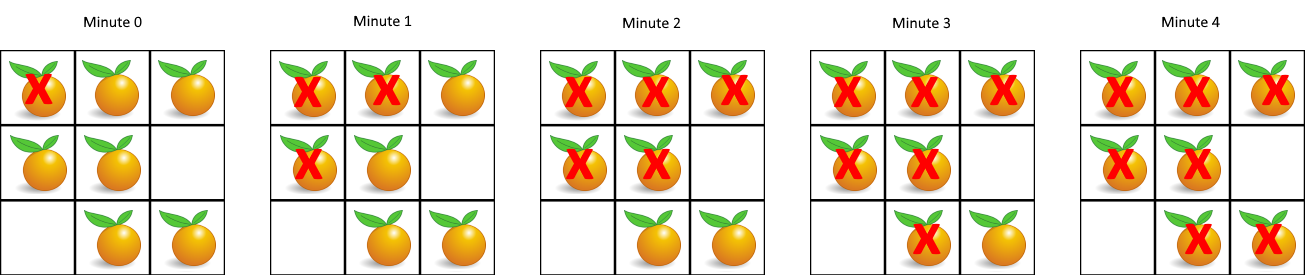
Input: [[2,1,1],[1,1,0],[0,1,1]] Output: 4
Example 2:
Input: [[2,1,1],[0,1,1],[1,0,1]] Output: -1 Explanation: The orange in the bottom left corner (row 2, column 0) is never rotten, because rotting only happens 4-directionally.
Example 3:
Input: [[0,2]] Output: 0 Explanation: Since there are already no fresh oranges at minute 0, the answer is just 0.
Note:
1 <= grid.length <= 10
1 <= grid[0].length <= 10
grid[i][j]
is only0
,1
, or2
.
Solution: BFS
Time complexity: O(mn)
Space complexity: O(mn)
C++
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 |
// Author: Huahua, Running time: 12 ms, 10.8 MB class Solution { public: int orangesRotting(vector<vector<int>>& grid) { const int m = grid.size(); const int n = grid[0].size(); queue<pair<int,int>> q; int fresh = 0; for (int i = 0; i < m; ++i) for (int j = 0; j < n; ++j) if (grid[i][j] == 1) ++fresh; else if (grid[i][j] == 2) q.emplace(j, i); vector<int> dirs = {1, 0, -1, 0, 1}; int days = 0; while (!q.empty() && fresh) { int size = q.size(); while (size--) { int x = q.front().first; int y = q.front().second; q.pop(); for (int i = 0; i < 4; ++i) { int dx = x + dirs[i]; int dy = y + dirs[i + 1]; if (dx < 0 || dx >= n || dy < 0 || dy >= m || grid[dy][dx] != 1) continue; --fresh; grid[dy][dx] = 2; q.emplace(dx, dy); } } ++days; } return fresh ? -1 : days; } }; |