Given an array of integers target
. From a starting array, A
consisting of all 1’s, you may perform the following procedure :
- let
x
be the sum of all elements currently in your array. - choose index
i
, such that0 <= i < target.size
and set the value ofA
at indexi
tox
. - You may repeat this procedure as many times as needed.
Return True if it is possible to construct the target
array from A
otherwise return False.
Example 1:
Input: target = [9,3,5] Output: true Explanation: Start with [1, 1, 1] [1, 1, 1], sum = 3 choose index 1 [1, 3, 1], sum = 5 choose index 2 [1, 3, 5], sum = 9 choose index 0 [9, 3, 5] Done
Example 2:
Input: target = [1,1,1,2] Output: false Explanation: Impossible to create target array from [1,1,1,1].
Example 3:
Input: target = [8,5] Output: true
Constraints:
N == target.length
1 <= target.length <= 5 * 10^4
1 <= target[i] <= 10^9
Solution: Backwards Simulation
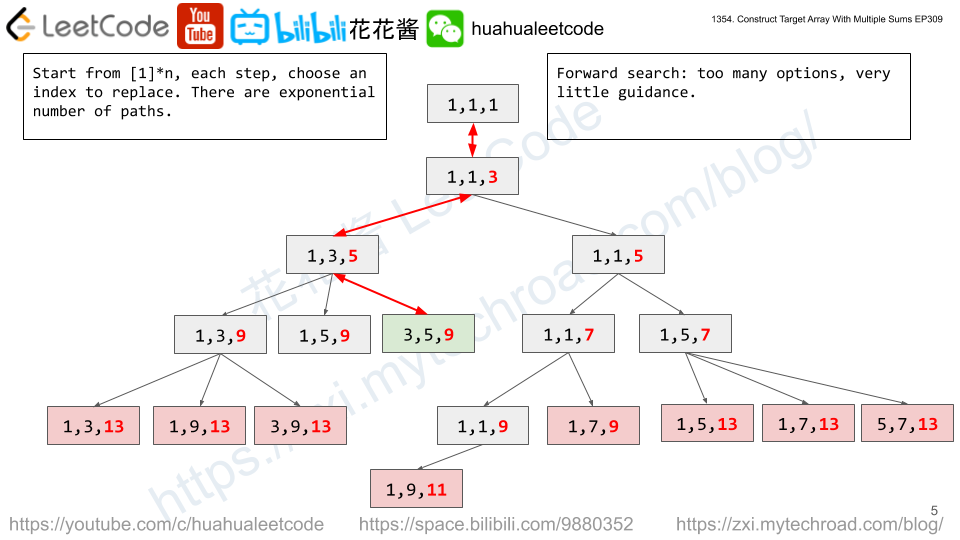
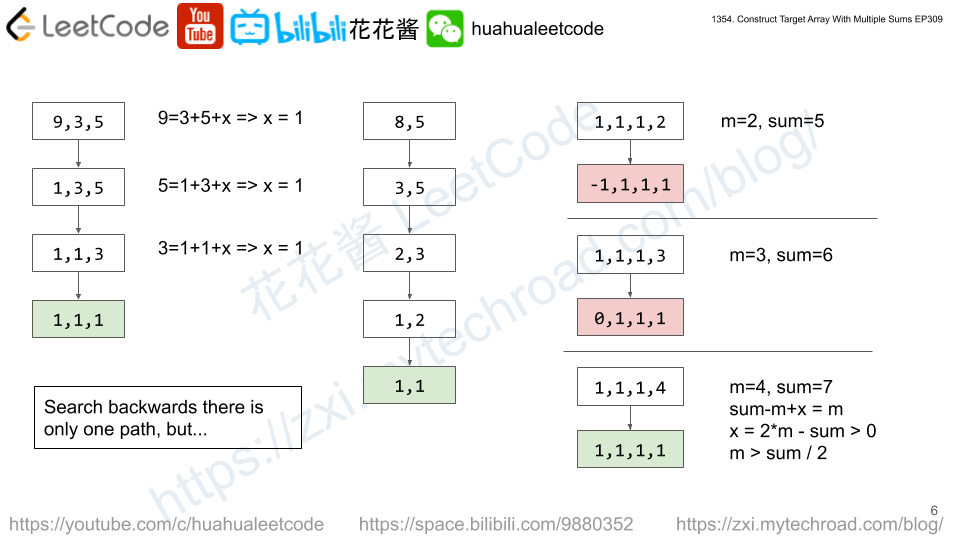
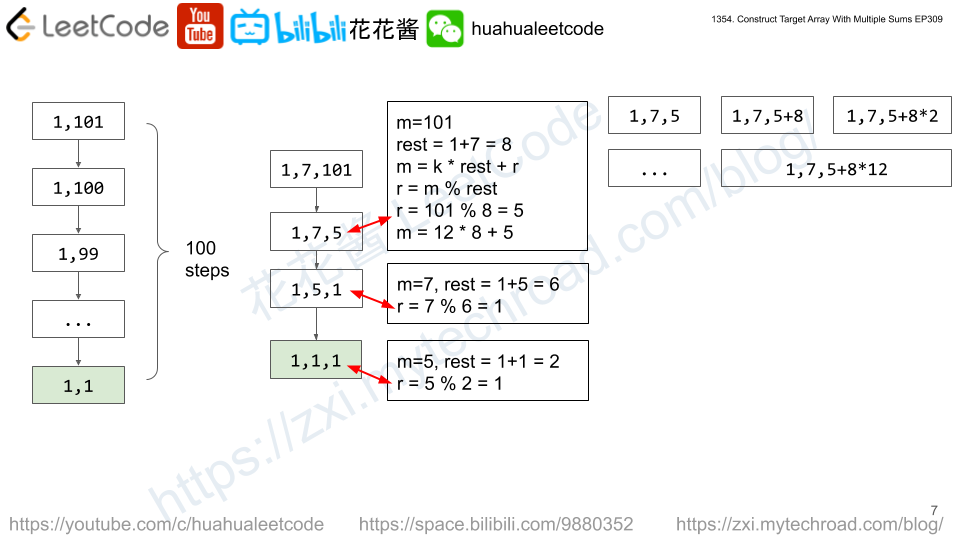
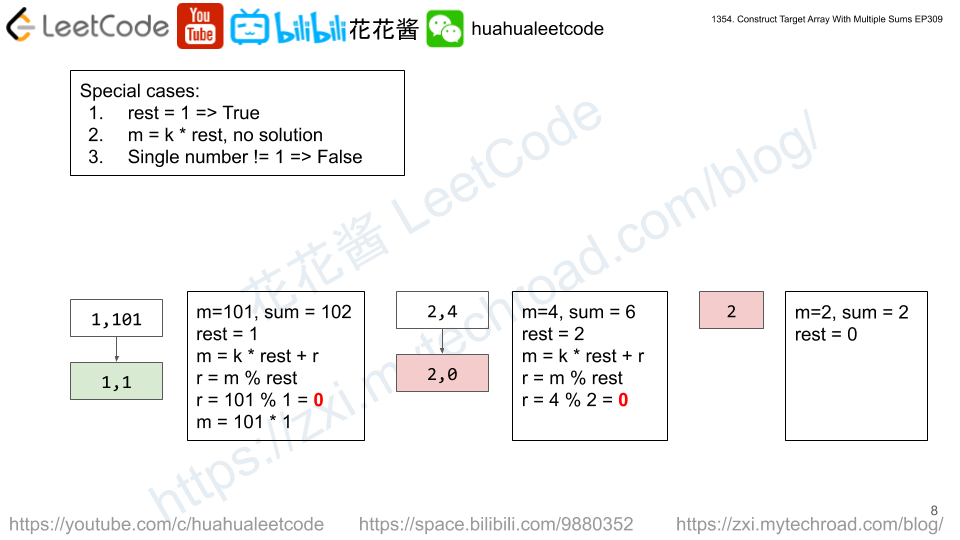
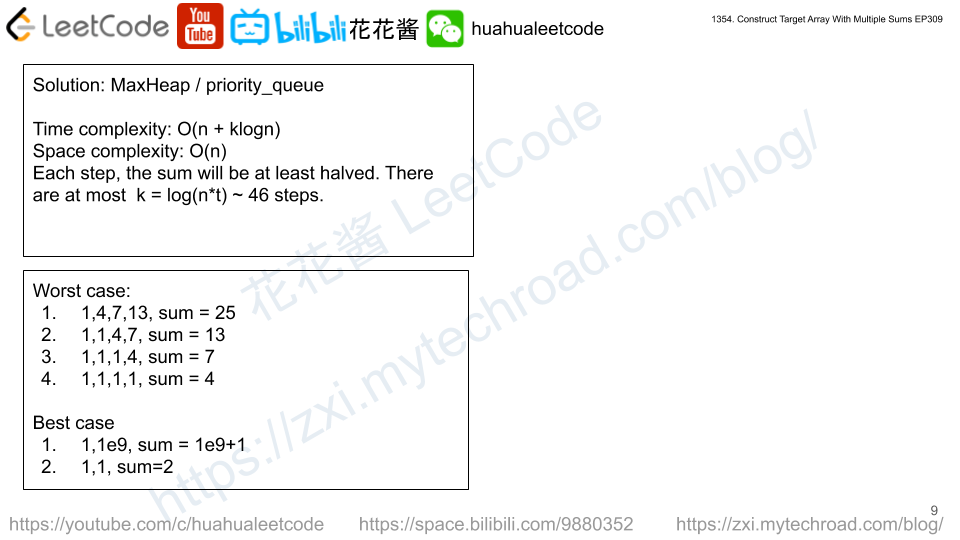
Start with the largest number in the array, since it should be the sum of all previous numbers.
[9,3,5] => [9 – (3+5), 3, 5] => [1, 3, 5] => [1, 3, 5 – (1+3)] => [1, 3, 1] => [1, 3 – (1+1), 1] => [1,1,1] done
Time complexity: O(n + log(n*t)*logn)
Space complexity: O(n)
C++
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
// Author: Huahua class Solution { public: bool isPossible(vector<int>& target) { priority_queue<int> q(begin(target), end(target)); long sum = accumulate(begin(target), end(target), 0LL); while (true) { int t = q.top(); q.pop(); sum -= t; if (t == 1 || sum == 1) return true; if (t < sum || sum == 0 || t % sum == 0) return false; t %= sum; sum += t; q.push(t); } return true; } }; |