A width x height
grid is on an XY-plane with the bottom-left cell at (0, 0)
and the top-right cell at (width - 1, height - 1)
. The grid is aligned with the four cardinal directions ("North"
, "East"
, "South"
, and "West"
). A robot is initially at cell (0, 0)
facing direction "East"
.
The robot can be instructed to move for a specific number of steps. For each step, it does the following.
- Attempts to move forward one cell in the direction it is facing.
- If the cell the robot is moving to is out of bounds, the robot instead turns 90 degrees counterclockwise and retries the step.
After the robot finishes moving the number of steps required, it stops and awaits the next instruction.
Implement the Robot
class:
Robot(int width, int height)
Initializes thewidth x height
grid with the robot at(0, 0)
facing"East"
.void move(int num)
Instructs the robot to move forwardnum
steps.int[] getPos()
Returns the current cell the robot is at, as an array of length 2,[x, y]
.String getDir()
Returns the current direction of the robot,"North"
,"East"
,"South"
, or"West"
.
Example 1:
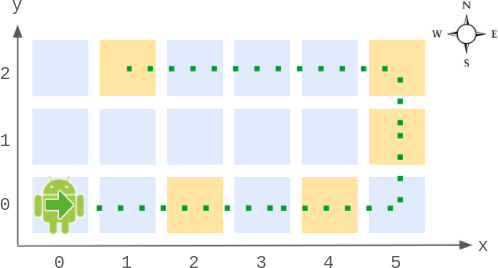
Input ["Robot", "move", "move", "getPos", "getDir", "move", "move", "move", "getPos", "getDir"] [[6, 3], [2], [2], [], [], [2], [1], [4], [], []] Output [null, null, null, [4, 0], "East", null, null, null, [1, 2], "West"] Explanation Robot robot = new Robot(6, 3); // Initialize the grid and the robot at (0, 0) facing East. robot.move(2); // It moves two steps East to (2, 0), and faces East. robot.move(2); // It moves two steps East to (4, 0), and faces East. robot.getPos(); // return [4, 0] robot.getDir(); // return "East" robot.move(2); // It moves one step East to (5, 0), and faces East. // Moving the next step East would be out of bounds, so it turns and faces North. // Then, it moves one step North to (5, 1), and faces North. robot.move(1); // It moves one step North to (5, 2), and faces North (not West). robot.move(4); // Moving the next step North would be out of bounds, so it turns and faces West. // Then, it moves four steps West to (1, 2), and faces West. robot.getPos(); // return [1, 2] robot.getDir(); // return "West"
Constraints:
2 <= width, height <= 100
1 <= num <= 105
- At most
104
calls in total will be made tomove
,getPos
, andgetDir
.
Solution: Simulation
Note num >> w + h, when we hit a wall, we will always follow the boundary afterwards. We can do num %= p to reduce steps, where p = ((w – 1) + (h – 1)) * 2
Time complexity: move: O(min(num, w+h))
Space complexity: O(1)
C++
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 |
// Author: Huahua constexpr static int dirs[4][2] = {{1, 0}, {0, 1}, {-1, 0}, {0, -1}}; const static vector<string> kNames{"East", "North", "West", "South"}; class Robot { public: Robot(int width, int height): w_(width), h_(height) { p_ = ((w_ - 1) + (h_ - 1)) * 2; } void move(int num) { while (num) { int tx = x_ + dirs[d_][0]; int ty = y_ + dirs[d_][1]; if (tx < 0 || tx >= w_ || ty < 0 || ty >= h_) { if (num > p_) num %= p_; if (num) d_ = (d_ + 1) % 4; continue; } x_ = tx; y_ = ty; --num; } } vector<int> getPos() { return {x_, y_}; } string getDir() { return kNames[d_]; } private: int w_; int h_; int d_{0}; // 0: E, 1: N, 2: W, 3: S int x_{0}; int y_{0}; int p_{0}; }; |
请尊重作者的劳动成果,转载请注明出处!花花保留对文章/视频的所有权利。
如果您喜欢这篇文章/视频,欢迎您捐赠花花。
If you like my articles / videos, donations are welcome.
Be First to Comment