You are given an integer matrix isWater
of size m x n
that represents a map of land and water cells.
- If
isWater[i][j] == 0
, cell(i, j)
is a land cell. - If
isWater[i][j] == 1
, cell(i, j)
is a water cell.
You must assign each cell a height in a way that follows these rules:
- The height of each cell must be non-negative.
- If the cell is a water cell, its height must be
0
. - Any two adjacent cells must have an absolute height difference of at most
1
. A cell is adjacent to another cell if the former is directly north, east, south, or west of the latter (i.e., their sides are touching).
Find an assignment of heights such that the maximum height in the matrix is maximized.
Return an integer matrix height
of size m x n
where height[i][j]
is cell (i, j)
‘s height. If there are multiple solutions, return any of them.
Example 1:
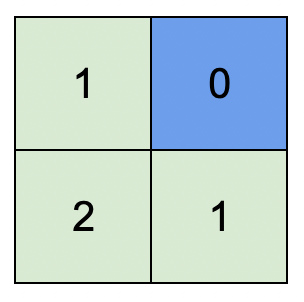
Input: isWater = [[0,1],[0,0]] Output: [[1,0],[2,1]] Explanation: The image shows the assigned heights of each cell. The blue cell is the water cell, and the green cells are the land cells.
Example 2:
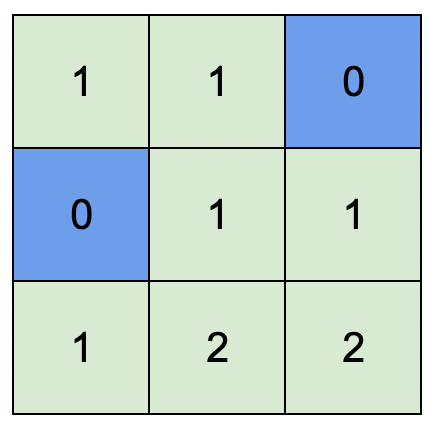
Input: isWater = [[0,0,1],[1,0,0],[0,0,0]] Output: [[1,1,0],[0,1,1],[1,2,2]] Explanation: A height of 2 is the maximum possible height of any assignment. Any height assignment that has a maximum height of 2 while still meeting the rules will also be accepted.
Constraints:
m == isWater.length
n == isWater[i].length
1 <= m, n <= 1000
isWater[i][j]
is0
or1
.- There is at least one water cell.
Solution: BFS
h[y][x] = min distance of (x, y) to any water cell.
Time complexity: O(m*n)
Space complexity: O(m*n)
C++
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
// Author: Huahua class Solution { public: vector<vector<int>> highestPeak(vector<vector<int>>& isWater) { const int m = isWater.size(); const int n = isWater[0].size(); vector<vector<int>> ans(m, vector<int>(n, INT_MIN)); queue<pair<int, int>> q; for (int y = 0; y < m; ++y) for (int x = 0; x < n; ++x) if (isWater[y][x]) { q.emplace(x, y); ans[y][x] = 0; } const vector<int> dirs{0, -1, 0, 1, 0}; while (!q.empty()) { const auto [cx, cy] = q.front(); q.pop(); for (int i = 0; i < 4; ++i) { const int x = cx + dirs[i]; const int y = cy + dirs[i + 1]; if (x < 0 || x >= n || y < 0 || y >= m) continue; if (ans[y][x] != INT_MIN) continue; ans[y][x] = ans[cy][cx] + 1; q.emplace(x, y); } } return ans; } }; |