You are given a 0-indexed 2D matrix grid
of size m x n
, where (r, c)
represents:
- A land cell if
grid[r][c] = 0
, or - A water cell containing
grid[r][c]
fish, ifgrid[r][c] > 0
.
A fisher can start at any water cell (r, c)
and can do the following operations any number of times:
- Catch all the fish at cell
(r, c)
, or - Move to any adjacent water cell.
Return the maximum number of fish the fisher can catch if he chooses his starting cell optimally, or 0
if no water cell exists.
An adjacent cell of the cell (r, c)
, is one of the cells (r, c + 1)
, (r, c - 1)
, (r + 1, c)
or (r - 1, c)
if it exists.
Example 1:
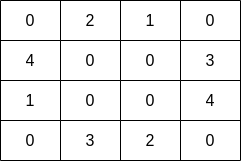
Input: grid = [[0,2,1,0],[4,0,0,3],[1,0,0,4],[0,3,2,0]] Output: 7 Explanation: The fisher can start at cell(1,3)
and collect 3 fish, then move to cell(2,3)
and collect 4 fish.
Example 2:
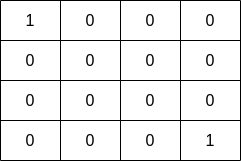
Input: grid = [[1,0,0,0],[0,0,0,0],[0,0,0,0],[0,0,0,1]] Output: 1 Explanation: The fisher can start at cells (0,0) or (3,3) and collect a single fish.
Constraints:
m == grid.length
n == grid[i].length
1 <= m, n <= 10
0 <= grid[i][j] <= 10
Solution: Connected Component
Similar to 花花酱 LeetCode 695. Max Area of Island
Find the connected component that has the max sum.
Time complexity: O(mn)
Space complexity: O(mn)
C++
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
// Author: Huahua class Solution { public: int findMaxFish(vector<vector<int>>& grid) { const int m = grid.size(); const int n = grid[0].size(); function<int(int, int)> dfs = [&](int r, int c) { if (r < 0 || r >= m || c < 0 || c >= n || !grid[r][c]) return 0; return exchange(grid[r][c], 0) + dfs(r - 1, c) + dfs(r, c - 1) + dfs(r + 1, c) + dfs(r, c + 1); }; int ans = 0; for (int r = 0; r < m; ++r) for (int c = 0; c < n; ++c) ans = max(ans, dfs(r, c)); return ans; } }; |