A city is represented as a bi-directional connected graph with n
vertices where each vertex is labeled from 1
to n
(inclusive). The edges in the graph are represented as a 2D integer array edges
, where each edges[i] = [ui, vi]
denotes a bi-directional edge between vertex ui
and vertex vi
. Every vertex pair is connected by at most one edge, and no vertex has an edge to itself. The time taken to traverse any edge is time
minutes.
Each vertex has a traffic signal which changes its color from green to red and vice versa every change
minutes. All signals change at the same time. You can enter a vertex at any time, but can leave a vertex only when the signal is green. You cannot wait at a vertex if the signal is green.
The second minimum value is defined as the smallest value strictly larger than the minimum value.
- For example the second minimum value of
[2, 3, 4]
is3
, and the second minimum value of[2, 2, 4]
is4
.
Given n
, edges
, time
, and change
, return the second minimum time it will take to go from vertex 1
to vertex n
.
Notes:
- You can go through any vertex any number of times, including
1
andn
. - You can assume that when the journey starts, all signals have just turned green.
Example 1:
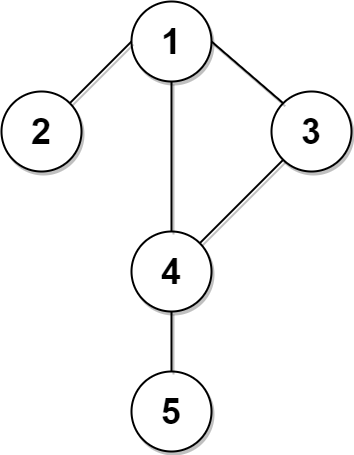
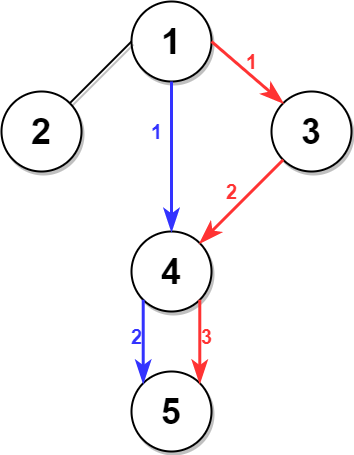
Input: n = 5, edges = [[1,2],[1,3],[1,4],[3,4],[4,5]], time = 3, change = 5 Output: 13 Explanation: The figure on the left shows the given graph. The blue path in the figure on the right is the minimum time path. The time taken is: - Start at 1, time elapsed=0 - 1 -> 4: 3 minutes, time elapsed=3 - 4 -> 5: 3 minutes, time elapsed=6 Hence the minimum time needed is 6 minutes. The red path shows the path to get the second minimum time. - Start at 1, time elapsed=0 - 1 -> 3: 3 minutes, time elapsed=3 - 3 -> 4: 3 minutes, time elapsed=6 - Wait at 4 for 4 minutes, time elapsed=10 - 4 -> 5: 3 minutes, time elapsed=13 Hence the second minimum time is 13 minutes.
Example 2:
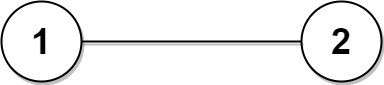
Input: n = 2, edges = [[1,2]], time = 3, change = 2 Output: 11 Explanation: The minimum time path is 1 -> 2 with time = 3 minutes. The second minimum time path is 1 -> 2 -> 1 -> 2 with time = 11 minutes.
Constraints:
2 <= n <= 104
n - 1 <= edges.length <= min(2 * 104, n * (n - 1) / 2)
edges[i].length == 2
1 <= ui, vi <= n
ui != vi
- There are no duplicate edges.
- Each vertex can be reached directly or indirectly from every other vertex.
1 <= time, change <= 103
Solution: Best first search
Since we’re only looking for second best, to avoid TLE, for each vertex, keep two best time to arrival is sufficient.
Time complexity: O(2ElogE)
Space complexity: O(V+E)
C++
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
// Author: Huahua class Solution { public: int secondMinimum(int n, vector<vector<int>>& edges, int time, int change) { vector<vector<int>> g(n + 1); for (const auto& e : edges) { g[e[0]].push_back(e[1]); g[e[1]].push_back(e[0]); } priority_queue<pair<int, int>> q; // {-t, node} q.emplace(0, 1); int min_time = -1; vector<vector<int>> ts(n + 1); ts[1].push_back(0); while (true) { const int t = -q.top().first; const int u = q.top().second; if (u == n) { if (min_time == -1 || t == min_time) { min_time = t; } else { return t; } } q.pop(); for (const auto& v : g[u]) { const int tt = (((t / change) & 1) ? (t + change - t % change) : t) + time; if ((ts[v].size() > 0 && tt == ts[v].back()) || ts[v].size() >= 2) continue; ts[v].push_back(tt); q.emplace(-tt, v); } } return -1; } }; |